Guidelines for Learning Python: A Clear and Confident Approach
Learning Python is becoming increasingly popular, and for good reason. Python is a versatile programming language used in various industries, including web development, data science, and machine learning. It is also known for its simplicity and readability, making it an excellent choice for beginners. However, like any new skill, learning Python can be a daunting task, especially for those who are new to programming.
To help those who are interested in learning Python, there are several guidelines that one can follow. These guidelines include finding a suitable learning resource, understanding the basics of Python, and practicing regularly. By following these guidelines, learners can gain a solid understanding of Python and its applications, and eventually become proficient in the language.
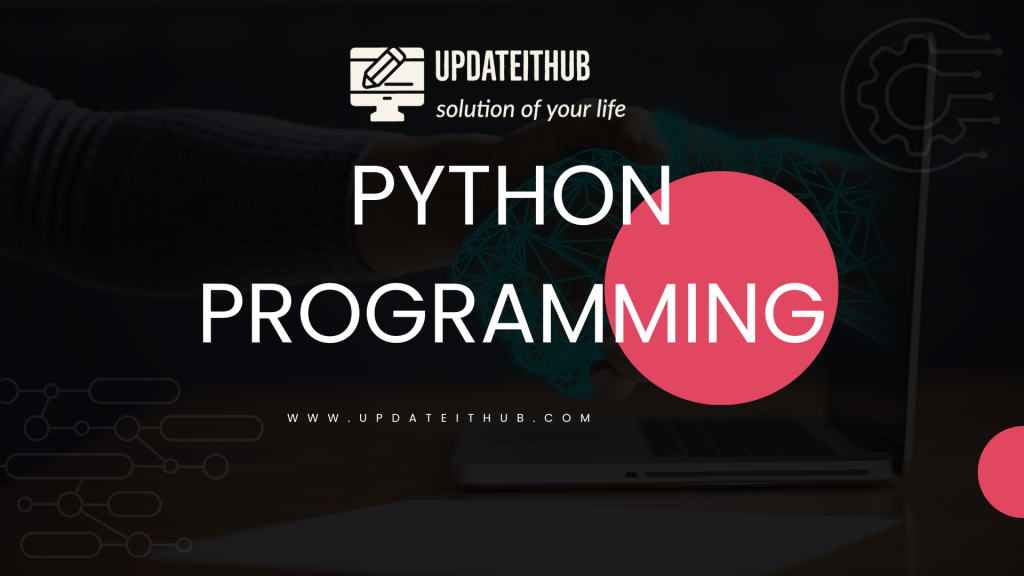
Setting Up the Python Environment
Before starting to learn Python, it is essential to set up the Python environment. This includes installing Python and any necessary packages and tools.
Installing Python
Python can be downloaded and installed from the official Python website, python.org. It is recommended to download the latest stable version of Python.
Virtual Environments
Virtual environments are used to create isolated Python environments for different projects. This helps to avoid conflicts between different versions of packages that may be required by different projects.
There are several tools available to create virtual environments, including virtualenv
and venv
. Both tools allow creating and managing virtual environments for Python projects.
Integrated Development Environment (IDE)
An Integrated Development Environment (IDE) is a software application that provides a comprehensive environment for coding, debugging, and testing Python code. There are several popular IDEs available for Python, including PyCharm, Visual Studio Code, and Spyder.
IDEs provide many features that help to improve productivity, such as code completion, debugging tools, and code profiling.
Package Management with pip
pip
is the default package manager for Python. It allows installing, upgrading, and managing Python packages.
When working on a project, it is recommended to create a requirements.txt
file that lists all the required packages for the project. This file can be used to install all the required packages in a new environment.
In conclusion, setting up the Python environment is an essential step before starting to learn Python. Installing Python, creating virtual environments, choosing an IDE, and using pip
for package management are some of the key considerations when setting up the environment.
Understanding Python Basics
Python is a popular programming language that is used extensively in various fields such as data science, artificial intelligence, web development, and more. Before diving into advanced topics, it is essential to understand the basics of Python. This section will cover the fundamental concepts of Python, including variables and data types, control structures, functions, and modules.
Variables and Data Types
Variables are used to store data in Python. In Python, you do not need to declare the type of the variable explicitly. Python automatically assigns the data type based on the value assigned to the variable. Python supports various data types, including integers, floating-point numbers, strings, Boolean, and more.
For example, to assign an integer value to a variable, you can use the following code:
x = 10
Similarly, to assign a string value to a variable, you can use the following code:
name = "John"
Control Structures
Control structures are used to control the flow of execution in a program. Python supports various control structures, including conditional statements and loops.
Conditional statements are used to execute a block of code if a particular condition is met. Python supports if-else statements and nested if-else statements. For example, the following code checks whether a number is positive, negative, or zero.
num = 10
if num > 0:
print("Positive Number")
elif num == 0:
print("Zero")
else:
print("Negative Number")
Loops are used to execute a block of code repeatedly. Python supports for and while loops. For example, the following code prints the numbers from 1 to 5 using a for loop.
for i in range(1, 6):
print(i)
Functions and Modules
Functions are used to group a set of related statements that perform a specific task. Python allows defining functions using the def keyword. For example, the following code defines a function that adds two numbers.
def add_numbers(a, b):
return a + b
Modules are used to organize code in Python. A module is a file containing Python code, and it can be imported into other Python programs. Python comes with many built-in modules, and you can also create your own modules. For example, the following code imports the math module and uses the sqrt function to calculate the square root of a number.
import math
num = 16
sqrt = math.sqrt(num)
print("Square root of", num, "is", sqrt)
Understanding the basics of Python is essential for anyone who wants to learn Python programming. By mastering the concepts of variables and data types, control structures, functions, and modules, you can start building more complex programs in Python.
Effective Python Practices
Python is a high-level programming language that is easy to learn and use. However, to become proficient in Python, it is important to follow effective practices that ensure code readability, error handling, and efficient use of libraries and frameworks.
Writing Readable Code
Writing readable code is essential for effective Python programming. It not only makes it easier for others to understand the code but also helps the programmer to maintain and update the code more efficiently.
Here are some tips for writing readable Python code:
- Use meaningful variable and function names that describe their purpose.
- Use comments to explain the code where necessary.
- Follow the PEP 8 style guide for Python code formatting.
- Use whitespace to separate code blocks and improve readability.
Error Handling
Python provides a robust error handling mechanism that allows programmers to catch and handle exceptions and errors that may occur during program execution.
Here are some tips for effective error handling in Python:
- Use try-except blocks to catch exceptions and handle errors.
- Use specific exception types to handle specific errors.
- Use logging to track errors and debug the code.
Using Libraries and Frameworks
Python has a vast collection of libraries and frameworks that can help programmers to build complex applications more efficiently.
Here are some tips for using libraries and frameworks in Python:
- Use popular libraries and frameworks that have a large community and good documentation.
- Read the documentation carefully to understand how to use the library or framework.
- Use virtual environments to manage dependencies and avoid conflicts between different libraries and frameworks.
By following these effective Python practices, programmers can write high-quality, efficient, and maintainable code that can be easily understood and updated by others.
Project-Based Learning
Python is a versatile programming language that can be used to build a variety of applications. Project-based learning (PBL) is a popular approach to learning Python as it allows learners to develop solutions to real-world problems and learn by doing. This section will discuss the key aspects of PBL for learning Python.
Selecting a Project
The first step in PBL is to select a project. Learners should choose a project that is of interest to them and relevant to their learning goals. It is important to select a project that is challenging but not too difficult. A project that is too easy may not provide enough learning opportunities, while a project that is too difficult may be overwhelming and demotivating.
Project Planning and Design
Once a project has been selected, learners should plan and design their project. This involves breaking the project down into smaller tasks and determining the order in which they will be completed. Learners should also consider the resources they will need, such as libraries, frameworks, and APIs. It is important to create a detailed plan and design as it will help learners stay on track and avoid getting stuck.
Implementation and Testing
The final step in PBL is implementation and testing. Learners should implement their project according to their plan and design. They should test their code regularly to ensure that it is working as expected and make any necessary changes. Learners should also seek feedback from others, such as peers and instructors, to improve their project.
In conclusion, PBL is a powerful approach to learning Python. By selecting a project, planning and designing it, and implementing and testing it, learners can develop real-world skills and gain valuable experience.
Advanced Python Concepts
Python is a versatile language that can be used for a variety of purposes. Once you have a good grasp of the basics, you can start exploring more advanced concepts that will allow you to take your Python skills to the next level. Here are some of the most important advanced Python concepts that you should consider learning:
Object-Oriented Programming
Python is an object-oriented language, which means that it has built-in support for classes and objects. Object-oriented programming (OOP) is a powerful programming paradigm that allows you to create reusable code and build complex applications. By using classes and objects, you can organize your code in a more logical and efficient way, making it easier to understand and maintain.
Decorators and Generators
Python has some powerful features that allow you to write more concise and expressive code. Decorators and generators are two such features. Decorators allow you to modify the behavior of functions or classes without changing their source code. Generators allow you to create iterators in a more efficient and elegant way. By mastering decorators and generators, you can write more efficient and expressive code.
Concurrency and Parallelism
Concurrency and parallelism are two important concepts that are essential for building high-performance applications. Concurrency is the ability to execute multiple tasks at the same time, while parallelism is the ability to execute multiple tasks simultaneously. Python has built-in support for concurrency and parallelism, which makes it an ideal language for building high-performance applications. By learning how to use concurrency and parallelism in Python, you can build applications that are faster and more efficient.
In conclusion, mastering advanced Python concepts can take your Python skills to the next level and allow you to build more complex and efficient applications. By learning about object-oriented programming, decorators and generators, and concurrency and parallelism, you can become a more skilled and versatile Python developer.
Staying Current with Python
Staying current with Python is essential for any programmer who wants to remain relevant in the field. Python is a dynamic language, and it is constantly evolving to meet the needs of its users. Here are a few tips to help you stay current with Python:
Following Python News
One of the best ways to stay current with Python is to follow news related to the language. There are many resources available for this purpose. For instance, the Python News section is home to official Python announcements, including releases, community news, and event listings. The most important Python developments are announced in the News section, so it is recommended to check it once a week (at least) to stay current.
Another way to stay up-to-date with Python is to subscribe to Python newsletters. For example, Python Weekly is a popular newsletter that provides a curated list of Python-related articles, news, and resources every week.
Participating in the Python Community
Participating in the Python community is another great way to stay current with Python. There are many ways to get involved in the community, such as attending Python conferences and events, joining Python user groups, and contributing to open-source Python projects.
Attending Python conferences and events is an excellent way to learn more about what other people or organizations are doing with Python. One such conference is PyCon, which is dedicated solely to the Python programming language. PyCon is held annually and attracts Python developers from all over the world.
Joining Python user groups is another way to participate in the community. User groups are local communities of Python enthusiasts who meet regularly to discuss Python-related topics. You can find a Python user group in your area by searching online.
Finally, contributing to open-source Python projects is a great way to learn more about Python and to improve your programming skills. There are many open-source Python projects available, and contributing to them can help you stay current with Python while also giving back to the community.
Frequently Asked Questions
What are the top resources for learning Python as a beginner?
Python is a widely used programming language, and there are many resources available online to help beginners learn it. Some of the top resources for learning Python as a beginner include Hackr.io, Educative.io, and the Python documentation. These resources offer a range of tutorials, courses, and FAQs to help beginners get started with Python.
How can I find practical Python coding exercises to enhance my skills?
One of the best ways to enhance your Python skills is to practice coding exercises. There are many websites that offer practical coding exercises, such as HackerRank and LeetCode. You can also find coding exercises in Python textbooks or online courses.
What are some recommended free e-books to help with learning Python?
Some recommended free e-books to help with learning Python include Think Python by Allen B. Downey, Automate the Boring Stuff with Python by Al Sweigart, and Python for Everybody by Charles Severance. These e-books cover the basics of Python programming and provide practical examples and exercises.
Can you suggest a structured step-by-step guide to learning Python?
A structured step-by-step guide to learning Python can be found on Python.org. This guide provides a comprehensive overview of Python programming, starting with the basics and progressing to more advanced topics. It also includes links to additional resources for further learning.
Where can I find Python coding examples to understand real-world applications?
Python is used in a variety of real-world applications, such as data analysis, web development, and machine learning. You can find Python coding examples on websites such as GitHub, Codecademy, and Kaggle. These websites offer a range of projects and examples to help you understand how Python is used in real-world applications.
What are the fundamental prerequisites before starting to learn Python?
Before starting to learn Python, it is recommended to have a basic understanding of programming concepts such as variables, data types, and control structures. It is also helpful to have some experience with another programming language, although this is not required. Additionally, having a strong foundation in mathematics can be beneficial for understanding more advanced Python concepts such as machine learning and data analysis.