Python Basics, Understanding Variables and Data Types
Python is a high-level, interpreted programming language that is widely used for developing web applications, scientific computing, and data analysis. Python is known for its simplicity, readability, and ease of use, making it an ideal language for beginners to learn. In this article, we will cover the basics of Python, including variables and data types.
Variables are an essential part of any programming language, and Python is no exception. A variable is a container that holds a value, which can be a number, a string, or any other data type. In Python, variables are created when a value is assigned to them. Unlike other programming languages, Python does not require you to declare the data type of a variable explicitly. Instead, Python infers the data type from the value that is assigned to the variable.
Python has several built-in data types, including numbers, strings, lists, tuples, and dictionaries. Each data type has its own unique properties and can be used to store different kinds of data. Understanding data types is essential in Python programming, as it allows you to manipulate data and perform various operations on it. In the next section, we will take a closer look at each data type and learn how to use them in Python.
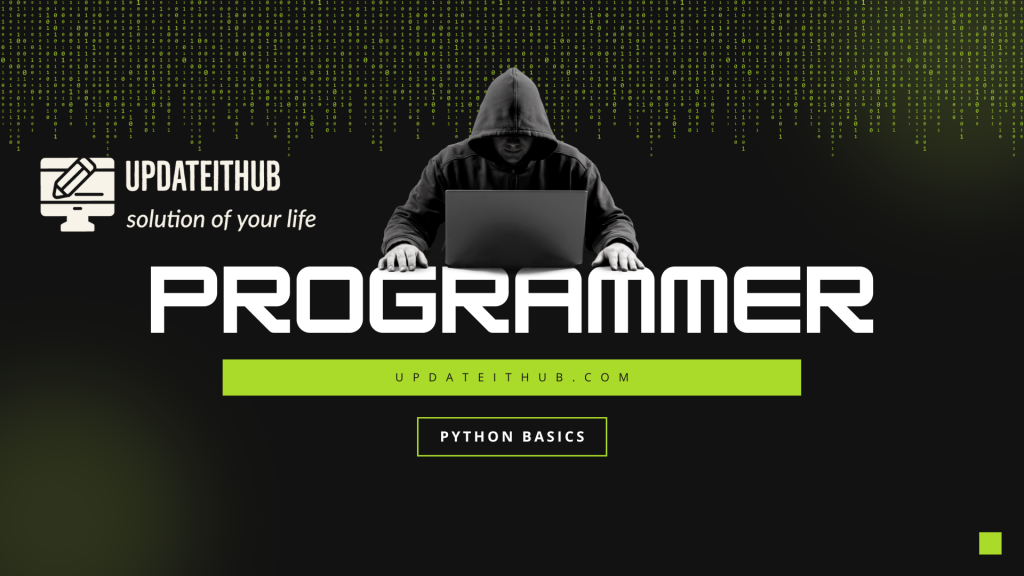
Getting Started with Python
Python is a popular programming language that is widely used in various fields, including web development, data science, machine learning, and more. In this section, we will cover the basics of Python, including installation and setup, and writing your first Python script.
Installation and Setup
Before you can start coding in Python, you need to install it on your computer. Python can be downloaded for free from the official website (https://www.python.org/downloads/) and is available for Windows, Mac, and Linux operating systems.
Once you have downloaded and installed Python, you can start coding in Python using a text editor or an Integrated Development Environment (IDE). Some popular text editors and IDEs for Python include Visual Studio Code, PyCharm, Sublime Text, and Atom.
Writing Your First Python Script
To get started with Python, let’s write a simple “Hello, World!” program. Open your text editor or IDE and create a new file. Type the following code:
print("Hello, World!")
Save the file with a .py extension, such as helloworld.py. Then, open a terminal or command prompt and navigate to the directory where you saved the file. Type the following command to run the program:
python helloworld.py
You should see the following output:
Hello, World!
Congratulations, you have written your first Python script! From here, you can start exploring the various data types, variables, and control structures that Python offers.
Understanding Python Syntax
Python is a high-level, interpreted programming language that is easy to learn and use. In this section, we will discuss the basic syntax rules of Python, including comments and documentation.
Basic Syntax Rules
Python syntax is simple and easy to learn. Here are some basic syntax rules that you should keep in mind while writing Python code:
- Python code is case-sensitive.
- Python statements should be written on separate lines. If you need to write a long statement, you can use the backslash () character to split the statement into multiple lines.
- Python does not use semicolons (;) to terminate statements.
- Python uses indentation to indicate blocks of code. The standard indentation is four spaces.
Comments and Documentation
Comments are used to explain the purpose of the code and to make it easier to read and understand. Python supports two types of comments: single-line comments and multi-line comments.
Single-line comments begin with the hash (#) character and continue until the end of the line. For example:
# This is a single-line comment
Multi-line comments are enclosed in triple quotes (”’ or “””) and can span multiple lines. For example:
'''
This is a multi-line comment.
It can span multiple lines.
'''
Documentation is used to provide information about the code, such as what it does, how to use it, and what parameters it takes. Python uses docstrings to provide documentation. A docstring is a string literal that appears as the first statement in a module, function, class, or method definition. For example:
def my_function(param1, param2):
"""
This function takes two parameters and returns their sum.
"""
return param1 + param2
In this example, the docstring provides information about the function, including what it does and what parameters it takes.
Working with Variables
Python variables are used to store data values. A variable is created as soon as a value is assigned to it. The equal sign (=) is used to assign values to variables.
Variable Assignment
In Python, variables can be assigned different types of data such as integers, floats, strings, and Boolean. The data type of a variable is determined automatically when a value is assigned to it. For example, the following code assigns an integer value to a variable named x
:
x = 5
This code assigns a string value to a variable named name
:
name = "John"
It is important to note that variable names cannot start with a number and cannot contain spaces. They can only contain letters, numbers, and underscores.
Variable Names
When naming variables, it is recommended to use descriptive names that reflect the purpose of the variable. This makes the code easier to read and understand. Python uses case-sensitive variable names, so age
and Age
are two different variables.
Python also has some reserved words that cannot be used as variable names, such as if
, else
, while
, and for
.
Overall, working with variables in Python is straightforward and flexible. By assigning values to variables, programmers can store and manipulate data in their programs.
Data Types in Python
Python has several built-in data types that are used to store different types of data. These data types can be broadly classified into two categories: Primitive Data Types and Collections.
Primitive Data Types
Primitive data types are the basic data types that are built into Python. These data types are immutable, which means that their value cannot be changed once they are created. The following are the primitive data types in Python:
- Integers: Integers are whole numbers, positive or negative, without decimals, of unlimited length. For example, 3, -5, 100, and 0 are all integers.
- Floats: Floats are numbers with decimal points. For example, 3.14, -0.5, and 1.0 are all floats.
- Booleans: Booleans are data types that can have one of two values: True or False. They are used to represent logical values. For example, the expression 2 + 2 == 4 evaluates to True, while the expression 2 + 2 == 5 evaluates to False.
- None: None is a special data type in Python that represents the absence of a value. It is often used to represent null or undefined values.
Collections
Collections are data types that are used to store collections of values. Unlike primitive data types, collections are mutable, which means that their values can be changed after they are created. The following are the collections data types in Python:
- Lists: Lists are used to store collections of values that are ordered and changeable. They are created using square brackets and can contain any data type. For example, [1, 2, 3], [“apple”, “banana”, “cherry”], and [1, “apple”, True] are all lists.
- Tuples: Tuples are used to store collections of values that are ordered and unchangeable. They are created using parentheses and can contain any data type. For example, (1, 2, 3), (“apple”, “banana”, “cherry”), and (1, “apple”, True) are all tuples.
- Sets: Sets are used to store collections of unique values that are unordered and unindexed. They are created using curly braces or the set() function and can contain any data type. For example, {1, 2, 3}, {“apple”, “banana”, “cherry”}, and {1, “apple”, True} are all sets.
- Dictionaries: Dictionaries are used to store collections of key-value pairs that are unordered and changeable. They are created using curly braces and can contain any data type. For example, {“name”: “John”, “age”: 30, “city”: “New York”} is a dictionary. In this example, “name”, “age”, and “city” are keys, while “John”, 30, and “New York” are values.
Control Structures
In Python, control structures are used to control the flow of execution of the program. Control structures are used to execute certain code blocks only if a certain condition is met or to repeat a code block multiple times. In this section, we will discuss two types of control structures: conditional statements and loops.
Conditional Statements
Conditional statements are used to execute a certain code block only if a certain condition is met. In Python, the if
statement is used to create a conditional statement. The if
statement is followed by a condition, and if the condition is true, the code block inside the if
statement is executed. If the condition is false, the code block inside the if
statement is skipped.
Python also supports else
and elif
statements. The else
statement is used to execute a code block if the condition in the if
statement is false. The elif
statement is used to create additional conditions to check if the first condition is false.
Here is an example of a conditional statement in Python:
x = 10
if x > 5:
print("x is greater than 5")
else:
print("x is less than or equal to 5")
Loops
Loops are used to repeat a code block multiple times. In Python, there are two types of loops: for
loops and while
loops.
The for
loop is used to iterate over a sequence of values. In Python, a sequence can be a list, a tuple, a string, or a range of numbers. The for
loop will execute the code block for each value in the sequence.
Here is an example of a for
loop in Python:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
The while
loop is used to repeat a code block as long as a certain condition is true. The condition is checked before each iteration of the code block.
Here is an example of a while
loop in Python:
i = 1
while i < 6:
print(i)
i += 1
In conclusion, control structures are an essential part of Python programming. They allow the programmer to control the flow of execution of the program and to repeat a code block multiple times. Conditional statements and loops are two types of control structures that are commonly used in Python.
Functions and Modules
Defining Functions
Functions in Python are blocks of code that perform a specific task. They are defined using the def
keyword followed by the function name and a set of parentheses containing any parameters the function requires. A colon is then used to indicate the beginning of the function block. The function block is indented and contains the code that performs the task.
def greet(name):
print("Hello, " + name + ". How are you doing today?")
In the above example, a function named greet
is defined that takes a single parameter name
. The function then prints a greeting message to the console that includes the value of the name
parameter. To call the function, simply pass a value for the name
parameter:
greet("John")
This will output: Hello, John. How are you doing today?
Importing Modules
Modules in Python are simply files containing Python definitions and statements. These files can be used to organize code and make it easier to reuse. To use a module in a Python program, it must first be imported using the import
statement.
import math
In the above example, the math
module is imported. This module contains many mathematical functions and constants, such as pi
and sqrt
. To use a function or constant from the module, simply prefix it with the module name:
print("The value of pi is approximately", math.pi)
This will output: The value of pi is approximately 3.141592653589793
It is also possible to import specific functions or constants from a module using the from
keyword:
from math import sqrt
print("The square root of 25 is", sqrt(25))
This will output: The square root of 25 is 5.0
Importing modules and functions is an important concept in Python programming, as it allows developers to reuse code and take advantage of existing libraries and frameworks.
File Handling
Python provides robust file handling capabilities that allow users to read from and write to files with ease. File handling in Python is highly flexible, as it allows you to work with different file types, including text files, binary files, CSV files, etc., and to perform different operations on files, including read, write, append, etc.
Reading Files
Reading files in Python is easy and straightforward. You can use the built-in open()
function to open a file and read its contents. The open()
function takes two arguments: the name of the file to open, and the mode in which to open the file. The mode can be set to read-only mode, write-only mode, or read-write mode.
Here is an example of how to read a file in Python:
with open('example.txt', 'r') as f:
data = f.read()
print(data)
In this example, the with
statement is used to open the file example.txt
in read-only mode. The contents of the file are then read using the read()
method, and stored in the variable data
. Finally, the contents of the file are printed to the console.
Writing to Files
Writing to files in Python is just as easy as reading from them. You can use the open()
function to open a file in write mode, and then use the write()
method to write data to the file.
Here is an example of how to write to a file in Python:
with open('example.txt', 'w') as f:
f.write('This is some example text.')
In this example, the with
statement is used to open the file example.txt
in write mode. The write()
method is then used to write the string 'This is some example text.'
to the file. When the with
statement is exited, the file is automatically closed.
Overall, file handling in Python is a powerful and flexible tool that can be used to read from and write to a wide variety of file types. By using the built-in open()
function and the various file handling methods available in Python, users can easily manipulate files to suit their needs.
Frequently Asked Questions
What are the different types of variables in Python?
In Python, there are four types of variables: integer, float, string, and boolean. An integer variable stores a whole number, a float variable stores a decimal number, a string variable stores a sequence of characters, and a boolean variable stores either True or False.
How do you define and use variables in Python?
To define a variable in Python, you simply assign a value to it using the equal sign (=). For example, to define an integer variable with a value of 5, you would write x = 5
. You can then use the variable in your code by referring to its name.
What are the core data types available in Python?
Python has several core data types, including integers, floats, strings, booleans, lists, tuples, sets, and dictionaries. Each data type has its own set of rules for how it can be used and manipulated.
Can you provide examples of how to work with string data types in Python?
Strings are used to store text in Python. You can define a string variable by enclosing text in either single quotes (”) or double quotes (“”). For example, name = "John"
defines a string variable called name
with a value of “John”. You can concatenate strings using the plus (+) operator, and access individual characters in a string using square brackets ([]).
How is the complex data type used in Python programming?
The complex data type in Python is used to represent complex numbers, which are numbers with both real and imaginary components. To define a complex variable, you use the syntax x = a + bj
, where a
is the real component and b
is the imaginary component. You can perform various operations on complex numbers, such as addition, subtraction, multiplication, and division.
What are the key differences between lists and other data structures in Python?
Lists are a type of data structure in Python that can store multiple values in a single variable. Lists are similar to arrays in other programming languages, but they have several key differences. For example, lists can store values of different data types, and they can be resized dynamically. Other data structures in Python include tuples, sets, and dictionaries, each with their own unique properties and use cases.