Python Functions and Dictionaries: A Comprehensive Guide
Python is a popular and versatile programming language that is widely used in various industries. One of the key features of Python is its ability to work with functions and dictionaries. Functions are blocks of code that can be called repeatedly to perform specific tasks, while dictionaries are collections of key-value pairs that allow for efficient data storage and retrieval.
In Python, functions are defined using the “def” keyword, followed by the function name and any parameters that are passed to it. Functions can be used to perform a wide range of tasks, from simple calculations to complex data processing operations. They are a powerful tool for organizing code and making it more modular, which can help to improve its readability and maintainability over time.
Dictionaries, on the other hand, are used to store and retrieve data in a way that is both efficient and intuitive. They are similar to lists in that they are collections of data, but they differ in that they use key-value pairs instead of indexes to access their elements. This makes them ideal for storing data that is organized in a non-sequential manner, such as contact information or product details. With their ability to store and retrieve data quickly, dictionaries are an essential tool for any Python programmer.
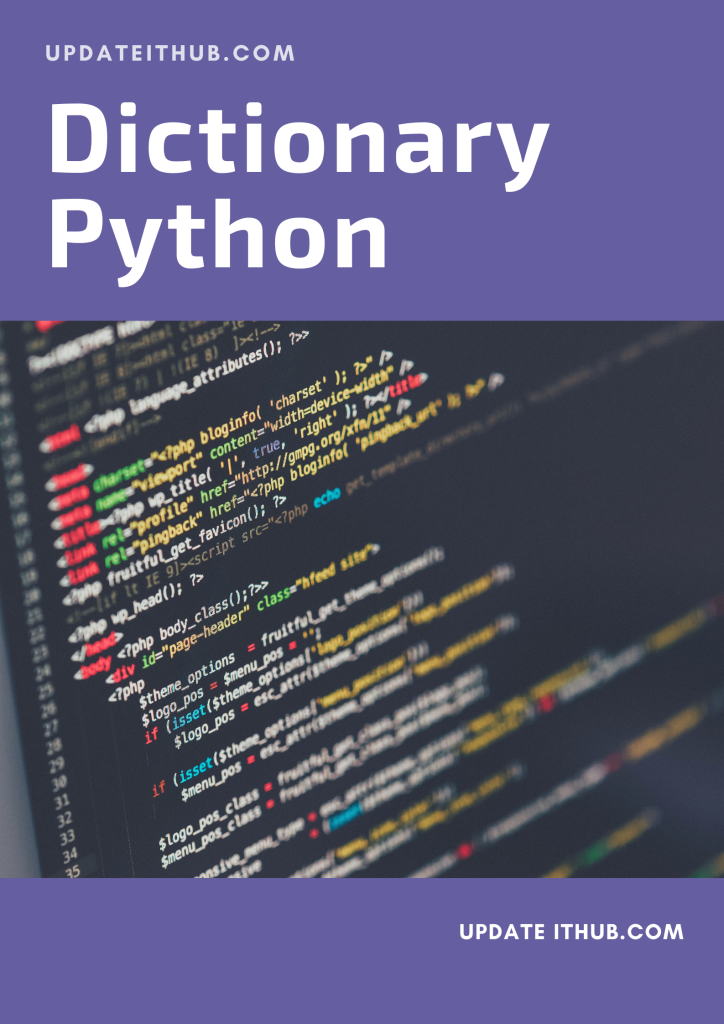
Understanding Python
Python is a high-level programming language that is widely used for web development, scientific computing, data analysis, artificial intelligence, and more. In this section, we will cover the basics of Python syntax, variables, and data types.
Python Syntax
Python has a simple and clean syntax that makes it easy to read and write code. Unlike other programming languages, Python does not use semicolons or curly braces to delimit blocks of code. Instead, Python uses indentation to indicate the level of nesting in the code.
For example, here is a simple Python program that prints “Hello, World!” to the console:
print("Hello, World!")
Python Variables
In Python, variables are used to store values that can be used later in the program. To create a variable in Python, you simply assign a value to a name using the equal sign. For example:
x = 42
In this example, the variable x
is assigned the value of 42
. Python is a dynamically typed language, which means that the type of a variable is determined at runtime based on the value that it holds.
Data Types in Python
Python has several built-in data types, including integers, floating-point numbers, strings, lists, tuples, and dictionaries.
- Integers: These are whole numbers, such as
42
or-10
. - Floating-point numbers: These are decimal numbers, such as
3.14
or-0.5
. - Strings: These are sequences of characters, such as
"Hello, World!"
or'Python is awesome!'
. - Lists: These are ordered collections of values, which can be of different types, such as
[1, 2, 3]
or["apple", "banana", "orange"]
. - Tuples: These are immutable ordered collections of values, which can be of different types, such as
(1, 2, 3)
or("apple", "banana", "orange")
. - Dictionaries: These are unordered collections of key-value pairs, such as
{"name": "John", "age": 30}
.
Understanding these basic concepts is essential for writing Python programs that are efficient, effective, and easy to read.
Python Functions
Functions are a fundamental concept in Python that allow developers to create reusable blocks of code. A function is a group of related statements that perform a specific task. Functions help to break down large programs into smaller, more manageable pieces, making code more modular and easier to read.
Defining Functions
In Python, a function is defined using the def
keyword, followed by the function name and a set of parentheses. The function definition can include parameters, which are values that the function expects to receive when it is called. The code that is executed when the function is called is enclosed in a block of code, which is indented under the function definition.
Function Parameters
Function parameters are values that are passed into a function when it is called. Parameters are defined in the function definition, and can be used to customize the behavior of the function. In Python, there are two types of function parameters: positional parameters and keyword parameters. Positional parameters are passed into the function in the order that they are defined, while keyword parameters are passed into the function using the parameter name.
Return Statement
The return
statement is used in Python functions to return a value to the caller. When a function is called, the code that is executed within the function can modify data or perform some other action, but the caller may also need to receive a value from the function. The return
statement allows the function to send a value back to the caller.
Scope and Lifetime of Variables
In Python, variables have a scope and a lifetime. The scope of a variable is the part of the program where the variable can be accessed, while the lifetime of a variable is the period of time during which the variable exists in memory. In Python, variables that are defined inside a function have a local scope, while variables that are defined outside a function have a global scope.
Anonymous Functions
In Python, anonymous functions are functions that are defined without a name. They are also known as lambda functions. Anonymous functions can take any number of arguments, but can only have one expression. They are often used as a shortcut to define small, one-time-use functions.
Python Dictionaries
Dictionaries in Python are a powerful and flexible data structure that allows you to store data in key-value pairs. They are similar to lists, but instead of using integers as indices, dictionaries use keys, which can be of any immutable type.
Creating Dictionaries
To create a dictionary, you can use curly braces {}
or the built-in dict()
function. Each key-value pair is separated by a colon :
and each pair is separated by a comma ,
. For example:
# Creating a dictionary using curly braces
person = {'name': 'John', 'age': 30, 'city': 'New York'}
# Creating a dictionary using the dict() function
person = dict(name='John', age=30, city='New York')
Accessing Dictionary Elements
To access a value in a dictionary, you can use the key as the index. For example:
person = {'name': 'John', 'age': 30, 'city': 'New York'}
print(person['name']) # Output: John
print(person['age']) # Output: 30
print(person['city']) # Output: New York
If the key is not found in the dictionary, a KeyError
will be raised. To avoid this, you can use the get()
method, which returns None
if the key is not found:
person = {'name': 'John', 'age': 30, 'city': 'New York'}
print(person.get('name')) # Output: John
print(person.get('gender')) # Output: None
Dictionary Methods
Python dictionaries have several built-in methods that allow you to manipulate the data they contain. Some of the most commonly used methods include:
keys()
: Returns a list of all the keys in the dictionary.values()
: Returns a list of all the values in the dictionary.items()
: Returns a list of all the key-value pairs in the dictionary as tuples.
Iterating Over Dictionaries
You can iterate over a dictionary using a for
loop. By default, the loop will iterate over the keys in the dictionary. For example:
person = {'name': 'John', 'age': 30, 'city': 'New York'}
for key in person:
print(key, person[key])
To iterate over the values in the dictionary, you can use the values()
method:
person = {'name': 'John', 'age': 30, 'city': 'New York'}
for value in person.values():
print(value)
To iterate over the key-value pairs in the dictionary, you can use the items()
method:
person = {'name': 'John', 'age': 30, 'city': 'New York'}
for key, value in person.items():
print(key, value)
Dictionary Comprehensions
Like lists, dictionaries can also be created using comprehensions. Dictionary comprehensions allow you to create a dictionary in a single line of code. For example:
# Creating a dictionary using a comprehension
squares = {x: x**2 for x in range(1, 6)}
print(squares) # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
In this example, a dictionary is created where the keys are the numbers 1 through 5 and the values are the squares of those numbers.
Frequently Asked Questions
How do you define and use functions in Python?
In Python, a function is defined using the def
keyword followed by the function name and parentheses. The function body is indented and contains the code to be executed when the function is called. To call a function, simply use the function name followed by parentheses and any required arguments.
What are the different ways to pass a dictionary to a function in Python?
There are two common ways to pass a dictionary to a function in Python. The first is to pass the dictionary as a parameter to the function, using the name of the dictionary as the parameter. The second is to use the **
operator to unpack the dictionary and pass it as keyword arguments to the function.
Can a Python dictionary store a function as a value, and how would you call it?
Yes, a Python dictionary can store a function as a value. To call the function, simply use the dictionary key to access the function and call it using parentheses.
What are the common methods available for dictionaries in Python?
Some of the common methods available for dictionaries in Python include clear()
, get()
, items()
, keys()
, and values()
. The clear()
method removes all the items from the dictionary, while the get()
method returns the value of a specified key. The items()
method returns a list of key-value pairs, while the keys()
method returns a list of all the keys in the dictionary. The values()
method returns a list of all the values in the dictionary.
How can you unpack a dictionary and pass it as keyword arguments to a function?
To unpack a dictionary and pass it as keyword arguments to a function, use the **
operator followed by the name of the dictionary. This will unpack the dictionary and pass its key-value pairs as keyword arguments to the function.
In what scenarios is it beneficial to use a dictionary with functions in Python programming?
Dictionaries are often used to store functions in Python programming when there is a need to map keys to functions. This is useful in scenarios where different functions need to be called based on user input or other conditions. Dictionaries with functions can also be used to implement switch-case statements in Python.