Python Programming, Working with Integers and Strings
Python is a popular programming language that is widely used for web development, data analysis, and artificial intelligence. One of the fundamental concepts in Python programming is understanding data types. Two of the most commonly used data types in Python are integers and strings. Integers are whole numbers that can be positive, negative, or zero, while strings are sequences of characters enclosed in quotation marks.
In Python programming, integers are used to represent numerical data. They can be used for performing mathematical operations such as addition, subtraction, multiplication, and division. Python provides several built-in functions for working with integers, such as abs(), pow(), and round(). Understanding how to work with integers is crucial for anyone learning Python programming, as they are used extensively in many applications. On the other hand, strings are used to represent text data. They can be used for storing and manipulating text, such as names, addresses, and messages. Python provides several built-in functions for working with strings, such as len(), upper(), and lower(). Understanding how to work with strings is also essential for anyone learning Python programming, as they are used extensively in many applications.
Python Programming Basics
Python is a high-level programming language that is widely used in web development, data analysis, artificial intelligence, and more. It is known for its simplicity, readability, and versatility. In this section, we will cover the basics of Python programming, including understanding Python syntax, setting up the Python environment, and writing your first Python script.
Understanding Python Syntax
Python uses a simple and easy-to-understand syntax that makes it a great language for beginners. One of the key features of Python is that it uses indentation to indicate blocks of code, instead of using braces like other programming languages. This makes the code easier to read and understand.
Python also has a number of built-in data types, including integers, strings, and lists. Integers are whole numbers, such as 1, 2, 3, and so on. They can be used in mathematical operations, such as addition, subtraction, multiplication, and division.
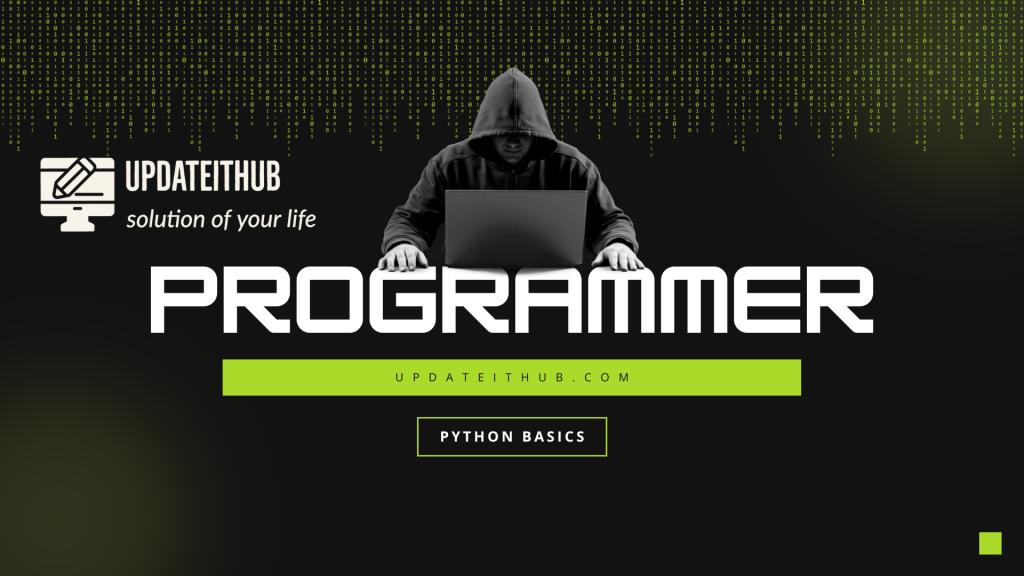
Setting Up the Python Environment
Before you can start programming in Python, you need to set up your environment. This involves installing Python on your computer and choosing an Integrated Development Environment (IDE) to work in. There are many different IDEs available for Python, including PyCharm, Visual Studio Code, and IDLE.
Once you have installed Python and chosen an IDE, you can start writing your first Python script. This involves opening a new file in your IDE and typing in your code. You can then save the file with a .py extension and run it from the command line.
Writing Your First Python Script
To get started with Python programming, you can write a simple “Hello, World!” program. This program simply prints the words “Hello, World!” to the screen. Here is an example of what the code would look like:
print("Hello, World!")
When you run this program, you should see the words “Hello, World!” printed to the screen.
In conclusion, Python programming is a powerful and versatile language that is widely used in many different fields. By understanding Python syntax, setting up your environment, and writing your first Python script, you can start exploring the many possibilities of this language.
Working with Integers in Python
In Python, integers are a fundamental data type and are used to represent whole numbers. They are immutable, which means that once they are created, they cannot be changed. This section will explore some of the ways in which integers can be used in Python.
Integer Operations
Python supports all of the standard arithmetic operations for integers, including addition, subtraction, multiplication, and division. In addition, Python also supports integer division, which returns the quotient of the division operation as an integer. This can be useful when working with large datasets or performing complex calculations.
Python also supports the modulo operator, which returns the remainder of the division operation. This operator can be used to determine whether a number is even or odd, or to perform cyclic operations.
Integer Functions
Python provides a number of built-in functions for working with integers. The abs()
function returns the absolute value of an integer, while the pow()
function can be used to raise an integer to a power. The round()
function can be used to round a floating-point number to the nearest integer.
Error Handling with Integers
When working with integers in Python, it is important to be aware of potential errors that can occur. One common error is a division by zero error, which occurs when attempting to divide an integer by zero. To handle this error, Python provides the ZeroDivisionError
exception.
Another common error is an overflow error, which occurs when attempting to perform a calculation that results in a number that is too large to be represented by an integer. To handle this error, Python provides the OverflowError
exception.
In conclusion, integers are a fundamental data type in Python and are used to represent whole numbers. Python provides a range of built-in operations and functions for working with integers, as well as error handling mechanisms to ensure that calculations are performed correctly.
String Manipulation in Python
Python is a popular programming language known for its simplicity and flexibility. It is widely used in various fields, including data science, web development, and artificial intelligence. One of the most important data types in Python is a string. A string is a sequence of characters, enclosed in single or double quotes. In this section, we will discuss how to manipulate strings in Python.
Basic String Operations
Python provides various basic operations to manipulate strings, such as concatenation, repetition, and slicing. Concatenation is the process of joining two or more strings together. It is performed using the +
operator. For example, "hello" + "world"
will result in "helloworld"
. Repetition is the process of repeating a string multiple times. It is performed using the *
operator. For example, "hello" * 3
will result in "hellohellohello"
. Slicing is the process of extracting a part of a string. It is performed using the []
operator. For example, "hello"[1:3]
will result in "el"
.
String Formatting
String formatting is the process of creating a formatted string by replacing placeholders with values. Python provides various ways to format strings, such as using the %
operator, the format()
method, and f-strings. The %
operator is used to replace placeholders with values. For example, "My name is %s and I am %d years old." % ("John", 25)
will result in "My name is John and I am 25 years old."
. The format()
method is used to replace placeholders with values, enclosed in curly braces {}
. For example, "My name is {} and I am {} years old.".format("John", 25)
will result in "My name is John and I am 25 years old."
. f-strings are a new way to format strings, introduced in Python 3.6. They are similar to using string literals, but with expressions inside curly braces. For example, f"My name is {'John'} and I am {25} years old."
will result in "My name is John and I am 25 years old."
.
String Methods and Functions
Python provides various built-in methods and functions to manipulate strings, such as len()
, upper()
, lower()
, strip()
, replace()
, and split()
. The len()
function is used to get the length of a string. For example, len("hello")
will result in 5
. The upper()
method is used to convert a string to uppercase. For example, "hello".upper()
will result in "HELLO"
. The lower()
method is used to convert a string to lowercase. For example, "HELLO".lower()
will result in "hello"
. The strip()
method is used to remove leading and trailing whitespace from a string. For example, " hello ".strip()
will result in "hello"
. The replace()
method is used to replace a substring with another substring. For example, "hello world".replace("world", "python")
will result in "hello python"
. The split()
method is used to split a string into a list of substrings, based on a delimiter. For example, "hello,world".split(",")
will result in ["hello", "world"]
.
Advanced Python Concepts
Python is a versatile programming language that can be used for a wide range of applications. In addition to its basic functionality, Python also offers several advanced concepts that can help developers create more complex and powerful programs. In this section, we will explore three of the most important advanced Python concepts: Object-Oriented Programming, Functional Programming, and Iterators and Generators.
Object-Oriented Programming
Object-Oriented Programming (OOP) is a programming paradigm that focuses on creating objects that have specific attributes and behaviors. In Python, OOP is implemented using classes and objects. A class is a blueprint for creating objects, while an object is an instance of a class.
Using OOP in Python can help developers create more modular and reusable code. By defining classes and objects, developers can encapsulate related data and functions, making it easier to manage and maintain their code.
Functional Programming
Functional Programming (FP) is another programming paradigm that is supported by Python. FP focuses on creating functions that are pure and do not have any side effects. This means that a function’s output depends only on its input, and it does not modify any external state.
Using FP in Python can help developers create more predictable and testable code. By creating pure functions, developers can avoid unexpected side effects and make it easier to reason about their code.
Iterators and Generators
Iterators and Generators are two advanced Python concepts that are related to each other. An iterator is an object that can be iterated over, while a generator is a special type of iterator that can be used to generate a sequence of values.
Using iterators and generators in Python can help developers create more memory-efficient and scalable code. By generating values on the fly, developers can avoid storing large amounts of data in memory, making their programs more efficient and faster.
In conclusion, Python offers several advanced concepts that can help developers create more powerful and flexible programs. By using OOP, FP, iterators, and generators, developers can create code that is easier to manage, maintain, and scale.
Best Practices in Python Programming
Python is a popular programming language that is known for its simplicity and ease of use. However, writing Python code that is efficient, readable, and maintainable requires following some best practices. This section will discuss some of the best practices that developers should follow when writing Python code.
Code Style and PEP 8
Code style is an important aspect of writing Python code. Following a consistent code style makes the code more readable and easier to maintain. The Python community has developed a style guide called PEP 8 that provides guidelines for writing Python code. PEP 8 covers topics such as naming conventions, indentation, and line length. Developers should follow PEP 8 when writing Python code to ensure that their code is consistent with the rest of the Python community.
Writing Efficient Python Code
Writing efficient Python code is important when working with large datasets or complex algorithms. One way to improve the performance of Python code is to use built-in functions and libraries. Python has a large standard library that includes many built-in functions and modules that can be used to perform common tasks. Using these built-in functions and modules can often be faster than writing custom code.
Another way to improve the performance of Python code is to use list comprehensions. List comprehensions are a concise way to create lists in Python. They are often faster than using traditional for loops because they are executed in C rather than Python.
Testing and Debugging
Testing and debugging are important parts of writing Python code. Developers should write unit tests to ensure that their code is working correctly. Unit tests are automated tests that check the functionality of individual units of code. They are often used to test functions and classes.
When debugging Python code, developers should use a debugger such as pdb. The pdb module provides a debugging environment for Python programs. It allows developers to step through code, inspect variables, and set breakpoints. Using a debugger can help developers quickly identify and fix bugs in their code.
In summary, following best practices when writing Python code can help developers write efficient, readable, and maintainable code. Developers should follow PEP 8 for code style, use built-in functions and libraries to improve performance, and write unit tests and use a debugger for testing and debugging.